Rules and languages
The Sonar Rules catalog is the entry point where you can discover all the existing rules. While running an analysis, SonarQube for IDE raises an issue every time a piece of code breaks a coding rule. Software quality classification and severity show the impact of the issue on your code.
See Software qualities for more information.
Overview
supported language versions
SonarQube for Visual Studio provides analysis for several languages. Support for your language may vary depending on the SonarQube for Visual Studio version you're running.
The table below lists the supported language and language versions. For each language version, the level of support is defined as follows:
- Fully supported: Analysis will complete. All the language features are understood and examined.
- Supported: Most language features are understood and examined but the version includes unsupported features. Analysis might break or provide incomplete results.
For language-specific properties, refer to the relevant language page in SonarQube Server, SonarQube Cloud, or SonarQube Community Build directly, as the same Sonar language analyzers are used by SonarQube for Visual Studio.
Supported out of the box: SonarQube for Visual Studio automatically checks your code in these languages and formats.
Connected Mode required: Running in Connected mode with SonarQube (Server, Cloud) or SonarQube Community Build unlocks analysis for these languages and formats.
Language | Supported version | |
---|---|---|
C rules | C89, C99, C11, C17: Fully supported C23: Supported GNU extensions: Supported | |
C# rules | C#1 to C#12: Fully supported | |
C++ rules | C++20, C++23: Supported C++03, C++11, C++14, C++17: Fully supported GNU extensions: Supported | |
CSS rules | Available from SonarLint for Visual Studio v6.16 and newer. | |
HTML | ||
JavaScript rules | ECMAScript 2022 and all previous versions: Supported | |
Secrets detection rules | ||
TypeScript rules | TypeScript 5.6 and all previous versions: Supported | |
T-SQL | ||
VB.NET rules | 7 to 16: Fully supported |
In addition, you will benefit from the following code analyzers: Sonar C#, Sonar VB.Net, Sonar C-Family for C or C++, and SonarJS.
For C# and VB.Net, new issues will be reported as you type. You do not have to select Run Code Analysis from the Analyze menu - the rules are run automatically. Note that by default, Visual Studio is configured to only run Roslyn analyzers on files that are currently open. You can choose to have the analysis run on the entire solution as described in the Microsoft docs, although this is obviously more processor-intensive.
For C, C++, JavaScript, and TypeScript, new issues will be reported when you open or save a file. Issues are highlighted in your code, and also listed in the 'Error List'.
You can access the detailed rule description directly from the issue in the Error List, using the Show Error help option on the contextual menu.
See the page on Connected mode for more details about language support for analyses while connected to SonarQube (Server, Cloud) or SonarQube Community Build.
Rule selection
The rules can be enabled and disabled locally. It is not currently possible to suppress individual issues. If you are using Connected mode with SonarQube (Server, Cloud) or SonarQube Community Build, the rule severities defined in the quality profile will be used.
Language-specific configuration
Define C# or VB.NET rules for local analysis
The Sonar C# and Sonar VB rules are implemented as Roslyn VSIX analyzers, and you can configure which rules are executed using the normal ruleset mechanism in VS.
How can I configure the C#/VB.NET rules to use?
SonarQube for Visual Studio itself does not have any special behavior in relation to configuring the set of Sonar C#/VB.NET rules. The Sonar C# and VB.NET rules are implemented as Roslyn analyzers and use the standard Visual Studio features to enable and disable rules.
For VS2015 and VS2017 this means ruleset files. VS2019+ users can use .editorconfig files instead.
The simplest approach is just to reference the ruleset directly from the project and check the ruleset file in to source control. This won't have any adverse impact on machines that don't have SonarQube for Visual Studio installed; Visual Studio will load the ruleset file, but it won't have any impact on build or in the IDE because the analyzers are not available.
How can I configure the C#/VB.NET rules to use without changing the project file or checking files in to source control?
There are a number of ways to achieve this, depending on the version of VS you are using. Some of the possible solutions are listed below. There may be others - see the Microsoft documentation Customize your build for more ideas.
1) Configure the rules in a .editorconfig file that is not under source control
The Visual Studio will search up the directory tree from the solution folder to locate a .editorconfig file. Create a .editorconfig file containing the required settings and drop it in a folder above the solution that is not under source control. See Set rule severity in an EditorConfig file in the Microsoft documentation for more information (setting a severity to "none" will disable a rule).
Limitations:
- this will only work in VS2019 or later
- it won't work if your solution already references a .editorconfig file
2) Add a Directory.Build.props file that references the ruleset file
The Directory.Build.props file will automatically be imported by MSBuild (and by extension Visual Studio). The file can be in the project directory or any parent directory so it should be possible to put it in a folder above the project/solution that is not under source code control.
Example:
<!-- Sample Directory.Build.props content -->
<Project>
<PropertyGroup>
<CodeAnalysisRuleSet>$(MSBuildThisFileDirectory)Example.ruleset</CodeAnalysisRuleSet>
</PropertyGroup>
</Project>
Limitations:
- this will only work on VS2017 or later.
- it won't work if your solution has a Directory.Build.props file checked in.
- it won't work if your solution already references a ruleset file.
3) Conditionally reference the ruleset file from the project, but don't check the ruleset file in to source control. The ruleset can be placed anywhere on your machine.
Example:
<!-- Snippet to conditionally set the ruleset to file that might not exist on all dev machines -->
<PropertyGroup>
<CodeAnalysisRuleset Condition="Exists('$(LocalAppData)\Example.ruleset')">$(LocalAppData)\Example.ruleset</CodeAnalysisRuleset>
</PropertyGroup>
Limitations:
- this does involve changing the project file(s), but only to add a single property.
4) Drop a props file that imports the ruleset file into the MSBuild ImportBefore folders
Project files that use the standard Microsoft build targets will automatically import any targets files that are exist in a well-known location. You can use this feature to conditionally import a ruleset.
This is similar to option (2) above but works with all versions of MSBuild.
Example:
<?xml version="1.0" encoding="utf-8"?>
<!-- Example props file to drop in %LocalAppData%\Microsoft\MSBuild\[VERSION]\Microsoft.Common.targets\ImportBefore -->
<Project xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<CodeAnalysisRuleset Condition="Exists('$(LocalAppData)\Example.ruleset')">$(LocalAppData)\Example.ruleset</CodeAnalysisRuleset>
</PropertyGroup>
</Project>
Notes:
- the location of the
ImportBefore
folder varies depending on the version of MSBuild, based on the following standard pattern:%LocalAppData%\Microsoft\MSBuild\[VERSION]\Microsoft.Common.targets\ImportBefore
. The[VERSION]
values for MSBuild 14 and 15 are14.0
and15.0
respectively. MSBuild 16 and latter useCurrent
. - the
ImportBefore
folder does not exist by default so you might have to create it.
Limitations:
- it won't work if your project already references a ruleset file.
- the location of the ImportBefore folder varies according to the version of MSBuild, so if you are using multiple versions of Visual Studio you will need to drop the same file in multiple locations.
Define C, C++, CSS, JS, TS or secrets detection rules for local analysis
In SonarLint for Visual Studio 7.3 and newer, changing the Sonar severity of an issue from the IDE is no longer possible. However, it is possible to Customize an issue's software quality severity level in SonarQube Server.
Enable or disable rule for local analysis
In standalone mode, it is possible to enable or disable rules, configure rule parameters, and in versions 4.13-7.2, change the reported rule severity. Not all options are supported for all languages. See the table below for more information:
Language | From version | Enable/disable | Change severity | Configure rule parameters |
---|---|---|---|---|
C | 4.13 | Supported | Supported in v4.13-7.2 | Supported |
C++ | 4.13 | Supported | Supported in v4.13-7.2 | Supported |
CSS | 8.0 | Supported | Not supported | Supported in v8.2 and newer |
JavaScript | 4.35 | Supported | Not supported. See #2399. | Supported in v8.2 and newer |
TypeScript | 4.35 | Supported | Not supported. See #2399. | Supported in v8.2 and newer |
Secrets detection | 6.4 | Supported | Not supported | Not applicable |
C# and VB.NET | 8.16 | Supported | Not supported | Supported |
Disabling a rule
To disable a rule, select an instance of the rule in the Error List, right-click on it, and choose the Disable rule command on the context menu:
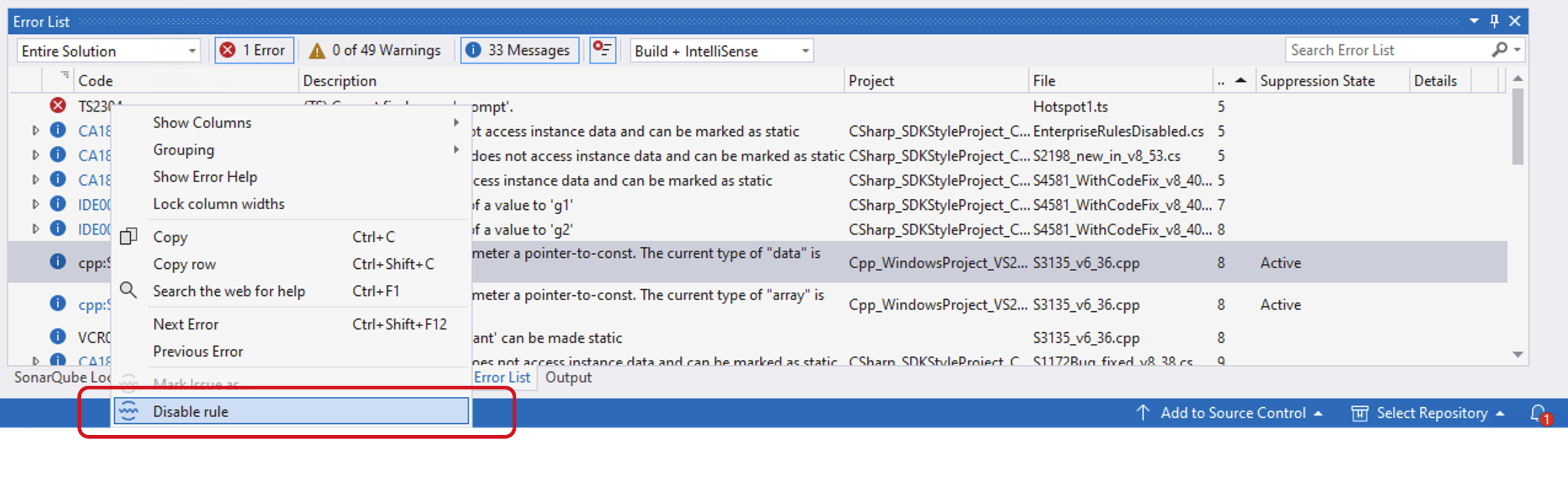
You can view and change the current rule settings by selecting Edit rules settings from the Extensions > SonarQube > Options window. Selecting Edit setting will open the settings.json file:
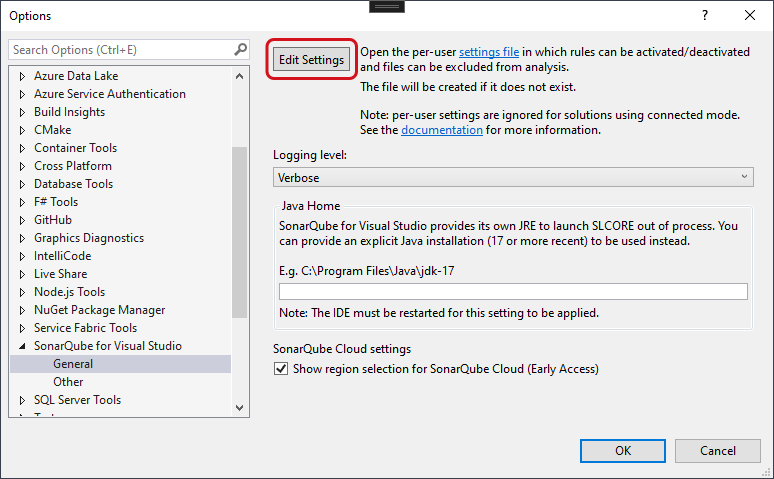
When the rule settings are changed (either by using the Disable rule command or by directly editing and saving the settings.json file, SonarQube for Visual Studio will automatically re-analyze all open documents.
The SonarQube for Visual Studio Output window tab will present a text output describing the processing that has taken place; see this example:
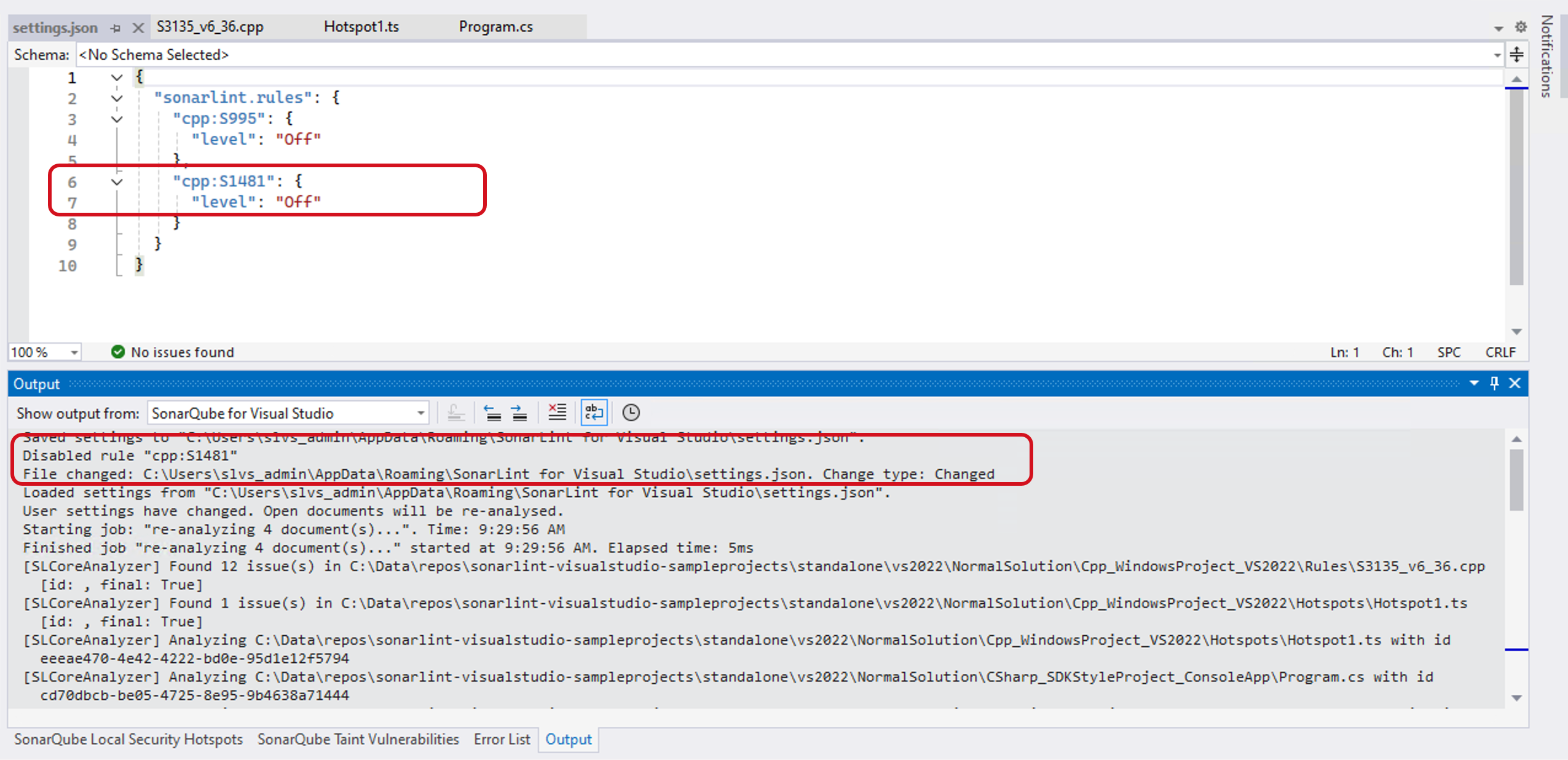
settings.json file format and location
The settings.json file is stored in your user's roaming profile %APPDATA%\SonarLint for Visual Studio
. It applies to all supported versions of Visual Studio. If the machine is domain-joined, then the settings file will be automatically copied to any other machine in the domain that the user logs on to.
See this example as a format sample of your settings.json file. It changes the parameters on a few rules, and at the end, excludes any files matching *Interface.cs
plus everything in the org/sonar/*
directory.
{
"sonarlint.rules": {
"c:S1135": {
"level": "On"
},
"cpp:S1199": {
"level": "Off"
},
"cpp:SingleGotoOrBreakPerIteration": {
"level": "On",
"parameters": {
"maxNumberOfTerminationStatements": "1"
}
},
"javascript:S1135": {
"level": "Off"
},
"typescript:S1854": {
"level": "On"
}
}
"sonarlint.analysisExcludesStandalone": "**/*Interface.cs,org/sonar/*"
}
The format of the rule key in the file is [c|cpp|javascript|typescript]:[rule id]
. The full list of rules is available at https://rules.sonarsource.com/.
If the settings file does not contain an entry for a rule then the default setting for the rule in the Sonar way Quality Profile will be used.
Note: the settings file is ignored when SonarQube for Visual Studio is running in Connected mode.
Sonar Rule Descriptions
SonarQube for Visual Studio can access descriptive and educational content associated with each issue. Simply select the issue’s rule, as shown below, to open the SonarQube Rule Help view and view the rule descriptions.
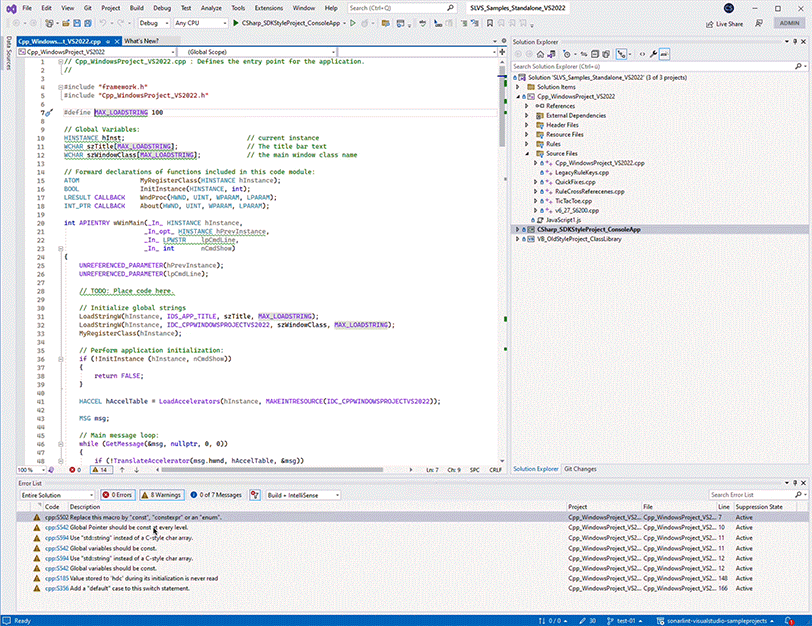
The SonarQube Rule Help view brings rule descriptions and patch instructions relevant to the library or framework you’re using, directly into the IDE. The rule descriptions include a brief explanation of the rule as well as Noncompliant and Compliant code samples.
Users can visualize a diff view for the non & compliant code samples, which should help you fix your issue. Note that diff highlighting is only available for rules descriptions migrated to the new format, and we're progressively migrating all existing rules to the new format.
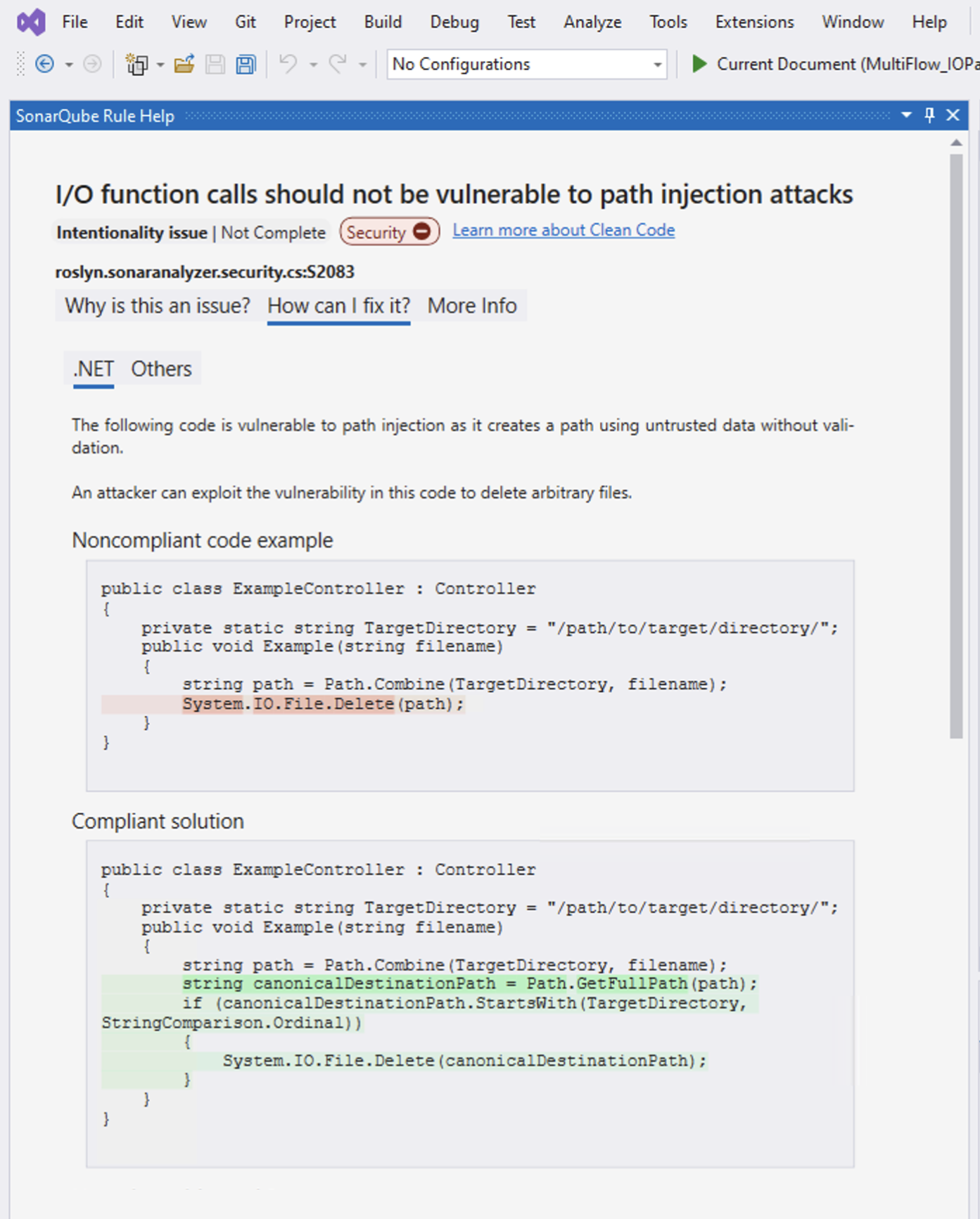
An issue’s Clean Code attribute, software qualities, and severity are presented to you when opening the SonarQube Rule Help view. Below the rule title, you will find the coding attribute labels that highlight an issue’s classification. Check the glossary for details about coding attributes, and the Software qualities page to better understand how they help classify your issue.
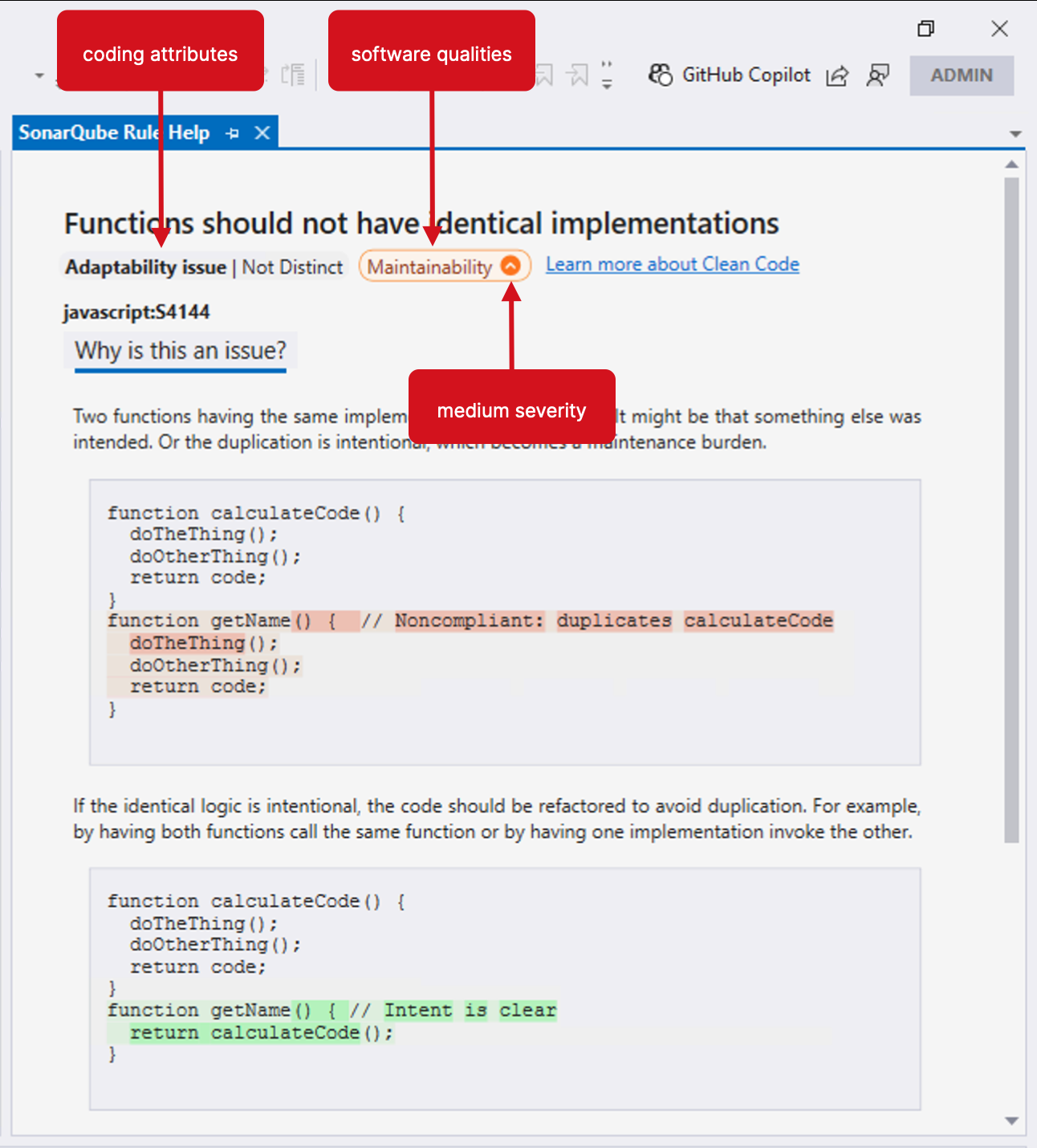
When in Connected Mode
If you’re running SonarQube for Visual Studio while in connected mode with SonarQube Server or SonarQube Community Build, your view will change according to the server settings. Standard Experience mode encompasses the use of rule types such as bugs, code smells, and vulnerabilities. Alternatively, if SonarQube Server is set to Multi-Quality Rule mode, you will more accurately represent the impact an issue has on all software qualities.
Please see the SonarQube Server and SonarQube Community Build articles for detailed information about the available rule modes.
Be sure to check out the Investigating issues page for more details about how issues appear in your IDE.
Applying rules while in Connected Mode
Connected mode syncs your SonarQube (Server, Cloud) or SonarQube Community Build quality profile with the local analysis to suppress issues reported in the IDE. See the Connected mode documentation for more information.
Rule severities
The Sonar rule severities defined by SonarQube (Server, Cloud) or SonarQube Community Build are different than the severities defined by Visual Studio. The mapping from Sonar severities to Visual Studio severities are as follows:
SonarQube Server/SonarCloud | Visual Studio |
Low | Message |
Medium | Warning |
High | Warning |
If you are using connected mode, the rule severities defined in the quality profile will be used.
In SonarLint for Visual Studio 7.3 and newer, changing the Sonar severity of an issue is not possible.
Other rule types
DBD rules
Dataflow bugs are what Sonar calls a series of advanced Python and Java bugs using symbolic execution. This type of issue can cause runtime errors and crashes in Python and Java. If you want to learn more, check out our blog post for a good explanation with an example.
Dataflow Bug Detection (DBD) rules for Python and Java are supported in Commercial Editions of SonarQube Server. At this time, SonarQube for Visual Studio does not support DBD detection.
Secrets detection
Starting with v6.4, SonarLint for Visual Studio will detect and report hard-coded cloud secrets as issues.
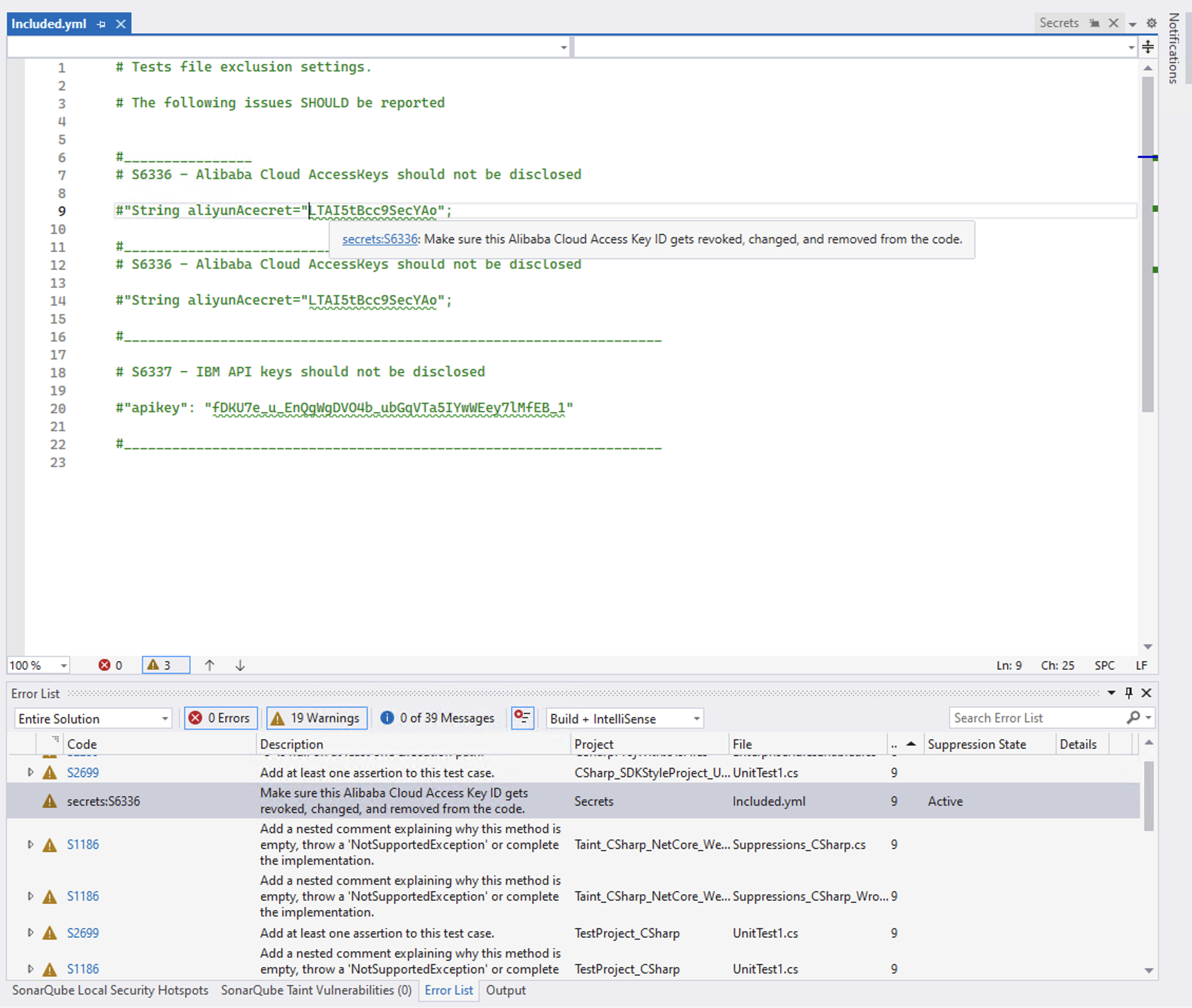
All types of text files are analyzed, irrespective of the type of content (code, configuration, documentation etc). Analysis is triggered whenever the file is opened or saved.
Documentation for individual Secrets rules can be found on the Rules website.
IDE-only
Secrets detection rules are only run in the IDE.
They do not appear in SonarQube (Server, Cloud) or SonarQube Community Build i.e. they can only be configured locally, and the secrets detection rules will not be run by the various Sonar scanners.
Was this page helpful?