Was this page helpful?
On this page
Clean Code definition
We define Clean Code as code that has the following attributes: consistency, intentionality, adaptability, and responsibility.
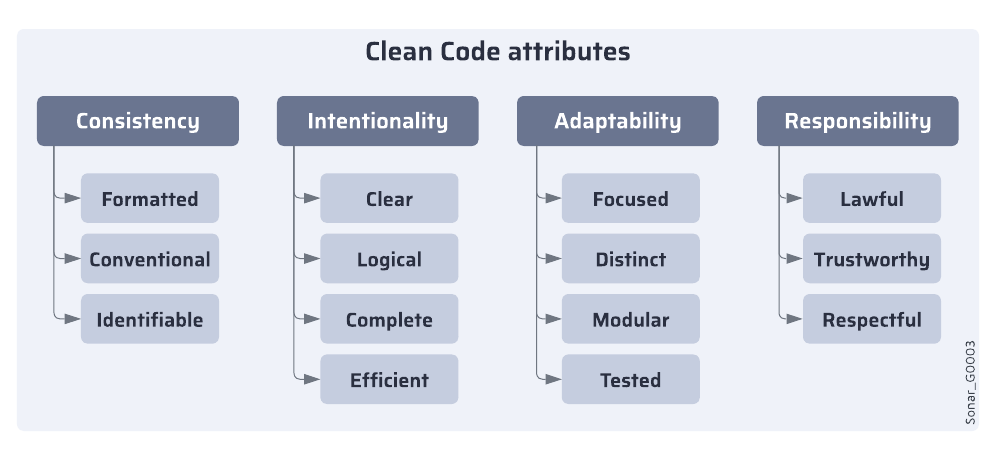
Consistency
The code is written in a uniform and conventional way. All the code looks similar and follows a regular pattern, even with multiple contributors at different times.
Consistent code is formatted, conventional, and identifiable.
Formatted
The code presentation is systematic and regular. Non-semantic choices, such as spacing, indentation, and character placement, remain consistent throughout the codebase, maintaining uniformity across files and authors.
Example
The example below shows inconsistent indentation in Java code. It’s not about tabs versus spaces, it’s about consistency.
Non-compliant code
class Foo {
public int a;
public int b;
public void doSomething() {
if(something) {
doSomethingElse();
}
}
}
Compliant code
class Foo {
public int a;
public int b;
public void doSomething() {
if(something) {
doSomethingElse();
}
}
}
For more information, see the corresponding Sonar rule.
Conventional
The code performs tasks with expected instructions. Faced with equally good options, the code adheres to a single choice across all instances, preferring language conventions. This includes using the appropriate programming interfaces and language features.
Example
In C++ from version 11, type aliases can be declared via either typedef
or using
, however, you should prefer the latter for modern code.
Non-compliant code
typedef void (*FunctionPointerType)(int);
Compliant code
using FunctionPointerType = void (*)(int);
For more information, see the corresponding Sonar rule.
Identifiable
The names follow a regular structure based on language conventions. The casing, word separators, suffixes, and prefixes used in the identifiers have purpose, without arbitrary differences.
Example
Consider code written in C#, where PascalCase is used for all identifiers except parameter names. In this context, using underscores or other casing styles to differentiate words in an identifier is unacceptable.
Non-compliant code
class my_class {...}
class SOMEName {...}
Compliant code
class MyClass {...}
class SomeName {...}
For more information, see the corresponding Sonar rule.
Intentionality
The code is precise and purposeful. Every instruction makes sense, is adequately formed, and clearly communicates its behavior.
Intentional code is clear, logical, complete, and efficient.
Clear
The code is self-explanatory, transparently communicating its functionality. It is written in a straightforward way that minimizes ambiguity, avoiding unnecessary clever or intricate solutions.
Example
In the non-compliant example of Python code below, and you'll notice that variables message
and i
are defined but never used. When readers encounter such cases, they might wonder if it's a coding error that was supposed to do something else or if it's just leftover code that can be safely deleted.
Non-compliant code
def hello(name):
message = "Hello " + name
print(name)
for i in range(10):
foo()
Compliant code
def hello(name):
message = "Hello " + name
print(message)
for _ in range(10):
foo()
For more information, see the corresponding Sonar rule.
Logical
The code has well-formed and sound instructions that work together. It is free of explicit errors, contradictions, and commands that could be unpredictable or objectionable.
Example
In JavaScript, there's NaN
, which stands for Not-a-Number. It represents a numeric data type that isn't a valid number. NaN
is not equal to any value, even itself, and this behavior can lead to unexpected results.
Non-compliant code
if (a !== NaN) {
console.log("this is always logged");
}
Compliant code
if (!isNaN(a)) {
console.log("a is not NaN");
}
For more information, see the corresponding Sonar rule.
Complete
The code constructs are comprehensive and used adequately and thoroughly. The code is functional and achieves its implied goals. There are no obviously incomplete or lacking solutions.
Example
An example in PHP is the use of secure cookies. The method setcookie
allows you to create cookies that can be transmitted via HTTP by default, making their contents readable. Since cookies often carry sensitive data, it's important to ensure they are transferred securely to fulfill their intended purpose. You need to pass a last argument to enable HTTPS only.
Non-compliant code
$value = "sensitive data";
setcookie($name, $value, $expire, $path, $domain);
Compliant code
$value = "sensitive data";
setcookie($name, $value, $expire, $path, $domain, true);
For more information, see the corresponding Sonar rule.
Adaptability
The code is structured to be easy to evolve and develop with confidence. It makes extending or repurposing its parts easy and promotes localized changes without undesirable side-effects.
Adaptable code is focused, distinct, modular, and tested.
Focused
The code has a single, narrow, and specific scope. Each unit should have only one concise purpose, without an overwhelming accumulation of instructions or excessive amounts of complexity.
Example
In Swift, it's best practice to keep types, such as classes, in separate files. This helps prevent an excessive accumulation of instructions or an overwhelming amount of complexity within a single file.
Non-compliant code
class MyViewController: UIViewController {
// …
}
extension MyViewController: UIScrollViewDelegate {
// …
}
class UnrelatedController: UIViewController {
// …
}
Compliant code
class MyViewController: UIViewController {
// …
}
extension MyViewController: UIScrollViewDelegate {
// …
}
For more information, see the corresponding Sonar rule.
Distinct
The code procedures and data are unique and distinctive, without undue duplication. The codebase has no significant repetition where it could be decomposed into smaller shared segments.
Example
Duplicating string literals raises the risk of errors when making updates since each occurrence must be changed separately. A better approach is to use constants that can be referenced from multiple places, allowing updates to be made in a single location. Here’s an example using Ruby.
Non-compliant code
def foo()
prepare('action random1')
execute('action random1')
release('action random1')
end
Compliant code
def foo()
action1 = 'action random1'
prepare(action1)
execute(action1)
release(action1)
end
For more information, see the corresponding Sonar rule.
Modular
The code has been organized and distributed to emphasize the separation between its parts. The relationships within the code are carefully managed, ensuring they are minimal and clearly defined.
Example
A key aspect of this is encapsulation. In Object-Oriented languages, encapsulation often involves making fields private. This way, the class retains control over the details of its internal representation and prevents other parts of the code from having too much knowledge about its inner workings.
However, there are multiple levels of encapsulation, and even minor improvements can make a difference. For example, if you're working with VB.Net, which allows publicly accessible fields, it's better to avoid using them and instead use properties. Properties work similarly to fields but are part of the interface and can be overridden by getters and setters.
Non-compliant code
Class Foo
Public Bar = 42
End Class
Compliant code
Class Foo
Public Property Bar = 42
End Class
For more information, see the corresponding Sonar rule.
Tested
The code has automated checks that provide confidence in the functionality. It has enough test coverage which enables changes in implementation without the risk of functional regressions.
Examples
There are odd examples, where you have a test folder or test files, without actual test cases inside, which can mislead other developers. See the corresponding Sonar rule.
There are also cases where tests are skipped and accidentally committed like that, which might go unnoticed if not tracked in any way. See the corresponding Sonar rule.
Responsibility
The code takes into account its ethical obligations on data, as well as societal norms.
Responsible code is lawful, trustworthy, and respectful.
Lawful
The code respects licensing and copyright regulation. It exercises the creator’s rights and honors other’s rights to license their own code.
Example
One common example is companies enforcing copyright headers in their code files:
/*
* SonarQube, open source software for clean code.
* Copyright (C) 2008-2024 SonarSource
* mailto:contact AT sonarsource DOT com
*
* SonarQube is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* SonarQube is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*/
For more information, see the corresponding Sonar rule.
Trustworthy
The code abstains from revealing or hard-coding private information. It preserves sensitive private information such as credentials and personally identifying information.
Example
Below is a simplified example using Go.
Non-compliant code
func connect() {
user := "root"
password:= "supersecret"
url := "login=" + user + "&passwd=" + password
}
Compliant code
func connect() {
user := getEncryptedUser()
password:= getEncryptedPass()
url := "login=" + user + "&passwd=" + password
}
For more information, see the corresponding Sonar rule.
Respectful
The code refrains from using discriminatory and offensive language. It chooses to prioritize inclusive terminology whenever an alternative exists that conveys the same meaning.
Example
Non-compliant code
Master / Slave
Blacklist / Whitelist
Compliant code
Primary / Secondary
Denylist / Allowlist
Learn more
Check the Clean Code benefits page to better understand software qualities. On the Code analysis based on Clean Code page you can learn how Clean Code attributes and software qualities are impacted by your code issue.